Static Factory Methods
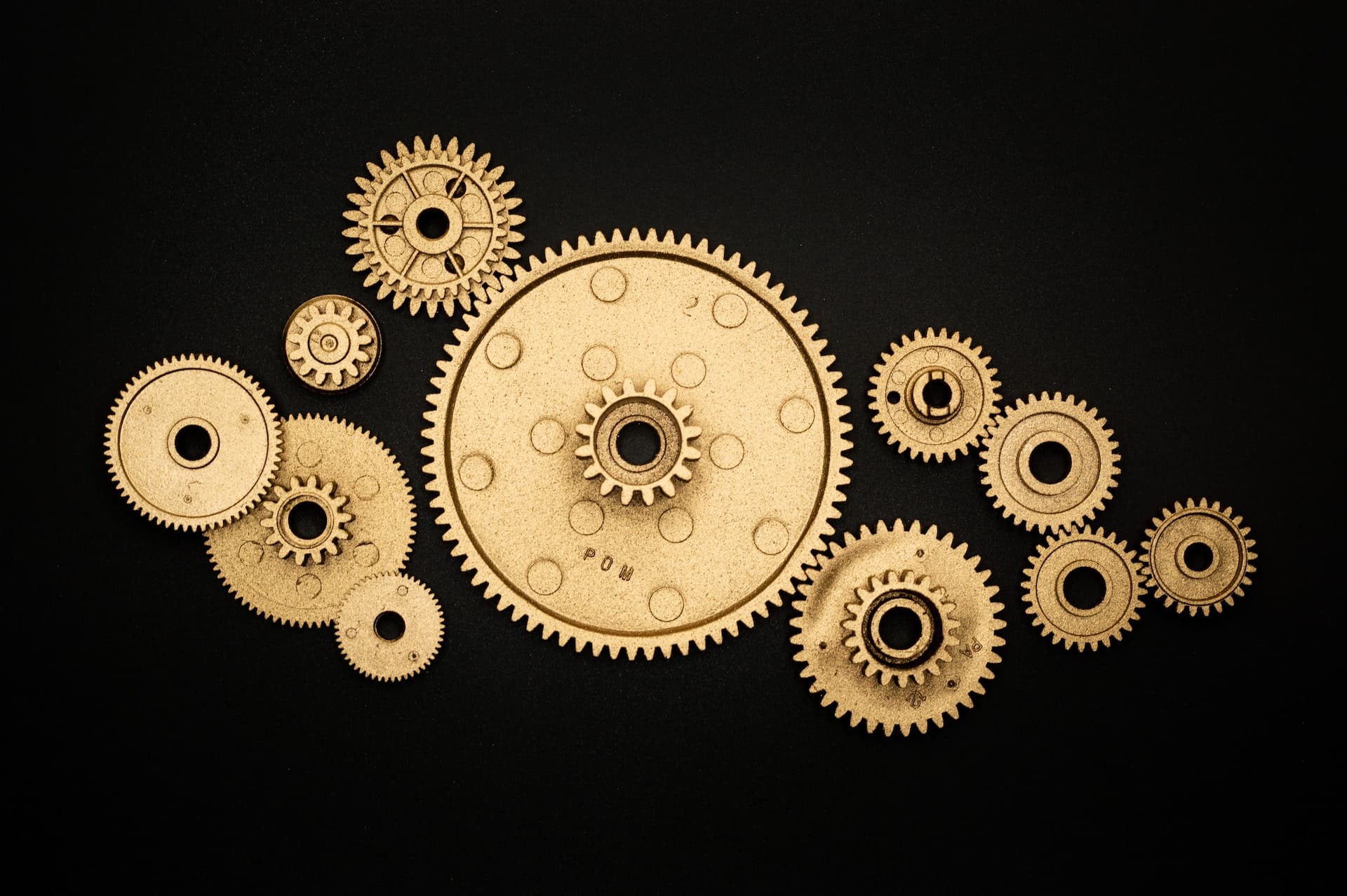
Using static factory methods instead of constructors can have several advantages
A class can provide a public static factory method instead of a public constructor to allow clients to obtain an instance. This has both advantages and disadvantages, but should be a part of every programmer's toolkit.
Advantages
Static factory methods can have names
Static factory methods are advantageous because they have names and can replace multiple constructors with the same signature, making code easier to read.
Static factory methods do not require to create a new object each time they're invoked
The advantages of static factory methods include their ability to return the same object from repeated invocations, and their ability to avoid creating unnecessary duplicate objects. This is especially useful for immutable classes, as it allows them to guarantee that no two equal instances exist. It also allows classes to be instance-controlled, which can be used to guarantee that they are singletons or noninstantiable, and to make sure that enums provide a guarantee of no two equal instances.
Static factory methods can return an object of any subtype of their return type
A third advantage of state factory methods is that, unlike constructors, they can return an object of any subtype of their return type. This gives flexibility in choosing the class of the returned object and allows API's to return objects without making their classes public, reducing the size and complexity of the API. As of Java 8, static members of an interface are required to be public, but private static methods are allowed in Java 9.
Static factory methods can return different types depending on the input parameters
Static factories provide the advantage of allowing the class of the returned object to vary from call to call as a function of the input parameters, and also from release to release. This makes it possible for implementations to change without affecting client code, which is beneficial for performance.
Static factory methods can return objects for which there exists no class yet
The fifth advantage of static factories is that the class of the returned object need not exist when the class containing the method is written. This is important in service provider frameworks such as the Java Database Connectivity API (JDBC), which have three essential components: a service interface, a provider registration API, and a service access API, which may allow clients to cycle through all available implementations. An optional fourth component is a service provider interface, which describes a factory object for producing instances of the service interface. Java 6 includes a general-purpose service provider framework, java.util.ServiceLoader, which should be used instead of writing one's own.
Disadvantages
If no constructors are uses, the class cannot be subclassed
Static factory methods limit the ability to subclass classes without public or protected constructors, but they can encourage composition over inheritance and are required for immutable types.
Static factory methods may are hard to find for programmers
Static factory methods are often preferred over public constructors as they are more concise and can be easier to find. Common names for these methods include "valueOf", "of", "getInstance", and "newInstance", and it is important to draw attention to them in API documentation.